Flask Hello World¶
An abbreviated tutorial to walk you through using the Flask library to build a web application better known as the beautiful giver of information, joy, data, and Nicolas Cage photos.
What is a “web application”¶
You’re familiar with a web browser. And perhaps you’re familiar with the idea that visiting a URL in a web browser serves up a web page. And maybe you think of https://www.nytimes.com
as the URL for the New York Times website. And the NYTimes website has many URLs that serve up many web pages, such as:
So what is a web application? For most intents and purposes to web visitors, a web application will feel like a web site in which going to a URL delivers a web page.
For example, you might know of the placecage.com site/web-application. Going to its homepage url at:
Renders this webpage (i.e a HTML file):
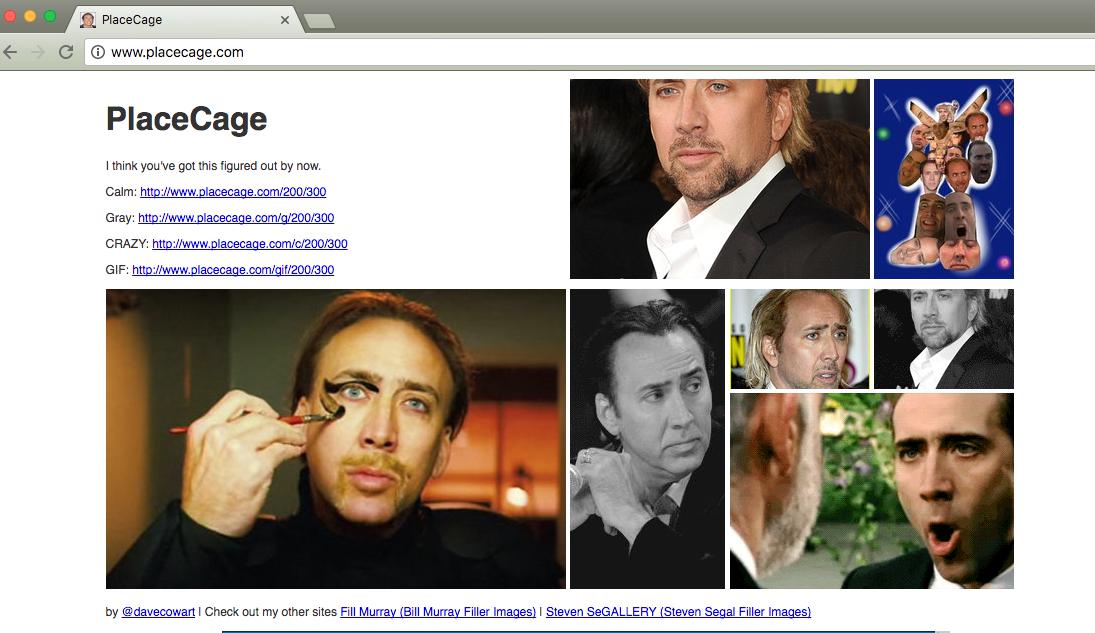
However, when we visit a different URL, such as:
The placecage.com web site – or, web app – renders an image that is 200
pixels wide by ``300``pixels high.
Changing up those values returns different images of different dimensions:
In other words, there seems to be a program behinc placecage.com that reacts – creates a Nicolas Cage image – when one of its URLs is visited v ia the web.
This program is a web application.
What is a news application¶
Think of “news application” as a fancy term for a web application that does something “newsy”.
News applications include such projects such as the Pulitzer-winning PolitiFact, or Chicago Tribune’s Crime in ChicagoLand (a descendant of one of the world’s first public data mashus, Adrian Holovaty’s chicagocrime.org) or ProPublica’s Dollars for Docs and Election DataBot are often described as news applications.
In the next section, we build a simple web application that does something when a URL is visited. It is up to you to modify it so that it is more of a news application, i.e. the one demonstrated in the excellent how-to-build-a-Flask-app-tutorial, First News App
Creating and running a local web application in Flask¶
We’re going to create a very simple Flask web application. Flask is the name of a Python framework for creating a web application. And if you’re on the Anaconda distribution, you should already have it.
A Python “application” being a “program”, this means ourFlask web application will be written in Python, saved as a Python script file, then executed.
Hello, local world¶
Web applications generally serve up HTML, which is the standard language for web pages. But I’ll assume we don’t even know what HTML is. As we’ve seen with placecage.com, a web app can serve up image bytes. And it probably follows logically that a web app can serve up plain text, like the string 'hello world'
The other thing that we associate with web apps is that they can be visited by the entire world, i.e. the “World” in World Wide Web. Does it seem like it will be complicated to make our simple Python script/application accessible to the whole world?
That’s...a whole other topic that we will ignore for now. Because we will run our web app locally. That means we can point a web browser on our own computer to a local URL that points to our local web application.
Easier to do than to fully explain:
The steps in this section will be a simplified version of this tutorial:
And we begin from scratch. No files, no created directories (yet). Just get your Atom (i.e. plaintext) editor open.
Creating a sub-directory named hello-flask
¶
Our web app will, initially, consist of one file and will live alone in a directory of its own. If you’ve been putting code at an easy to get directory, e.g. all of your homework scripts in ~/Downloads
– stop doing that.
Instead, make a sub-folder off of ~/Downloads
, e.g. Downloads/hello-flask
. In the examples below, I’m an OS X/Linux system, which has a /tmp
folder that I like to use because it gets wiped out when I restart my computer. So, my web-app home will be /tmp/hello-flask
, though the exact name really doesn’t matter.
Create this subdirectory however you make sub-directories on your computer.
Opening a directory as a project with Atom text edtiro¶
Next, using the Atom text editor, select the File > Open...
menu command, and target whatever directoryyou just created. In my example, I’m opening /tmp/hello-flask
:
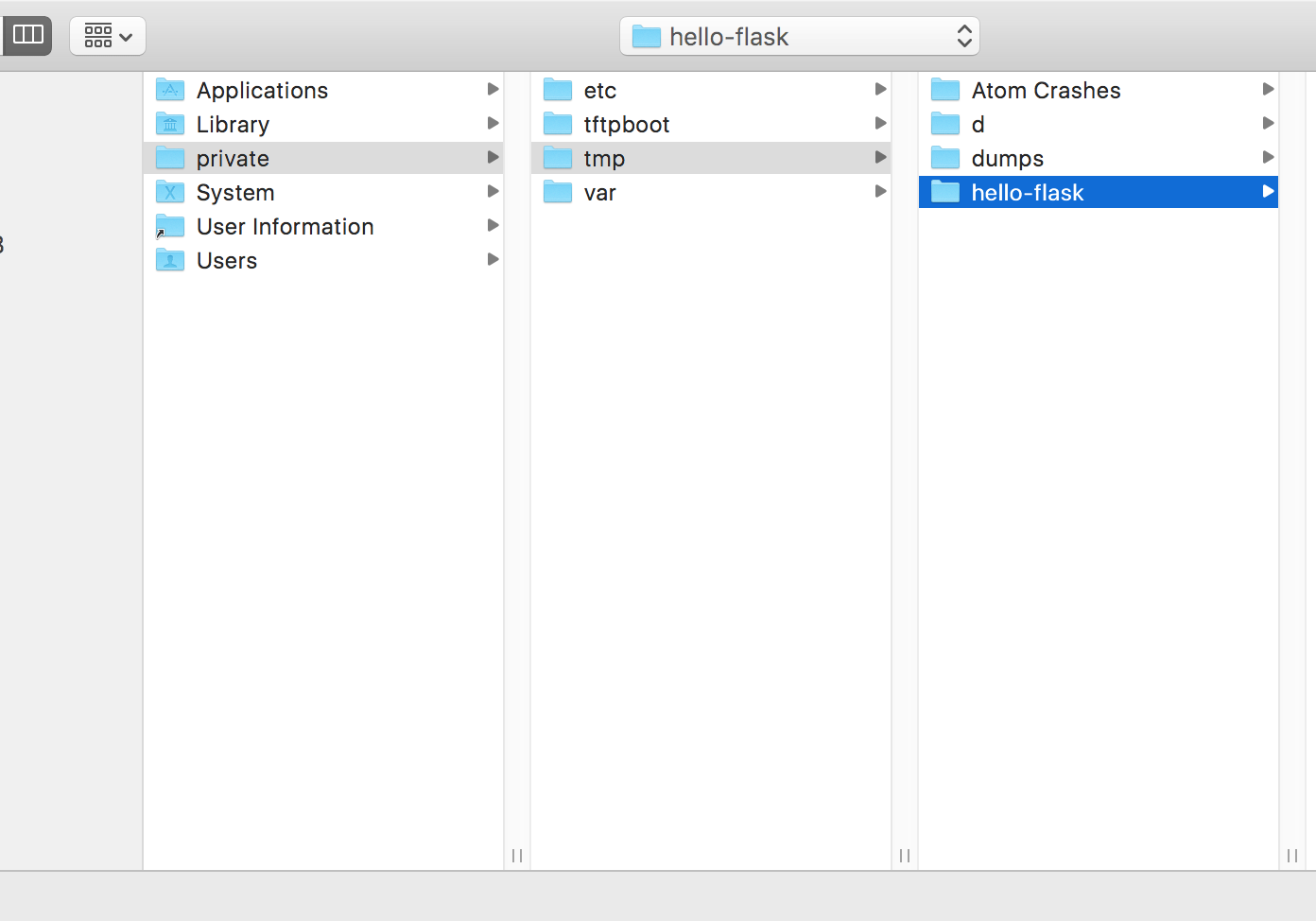
Note
Just the subdirectory, please
You should be opening hello-flask
only, not its parent directory, e.g. ~/Desktop/hello-flask
or /tmp/hello-flask
Opening an file folder should result in Atom’s project sidebar showing up on the right-side of the Atom editor. Because my /tmp/hello-flask
folder is empty, my project sidebar is empty:
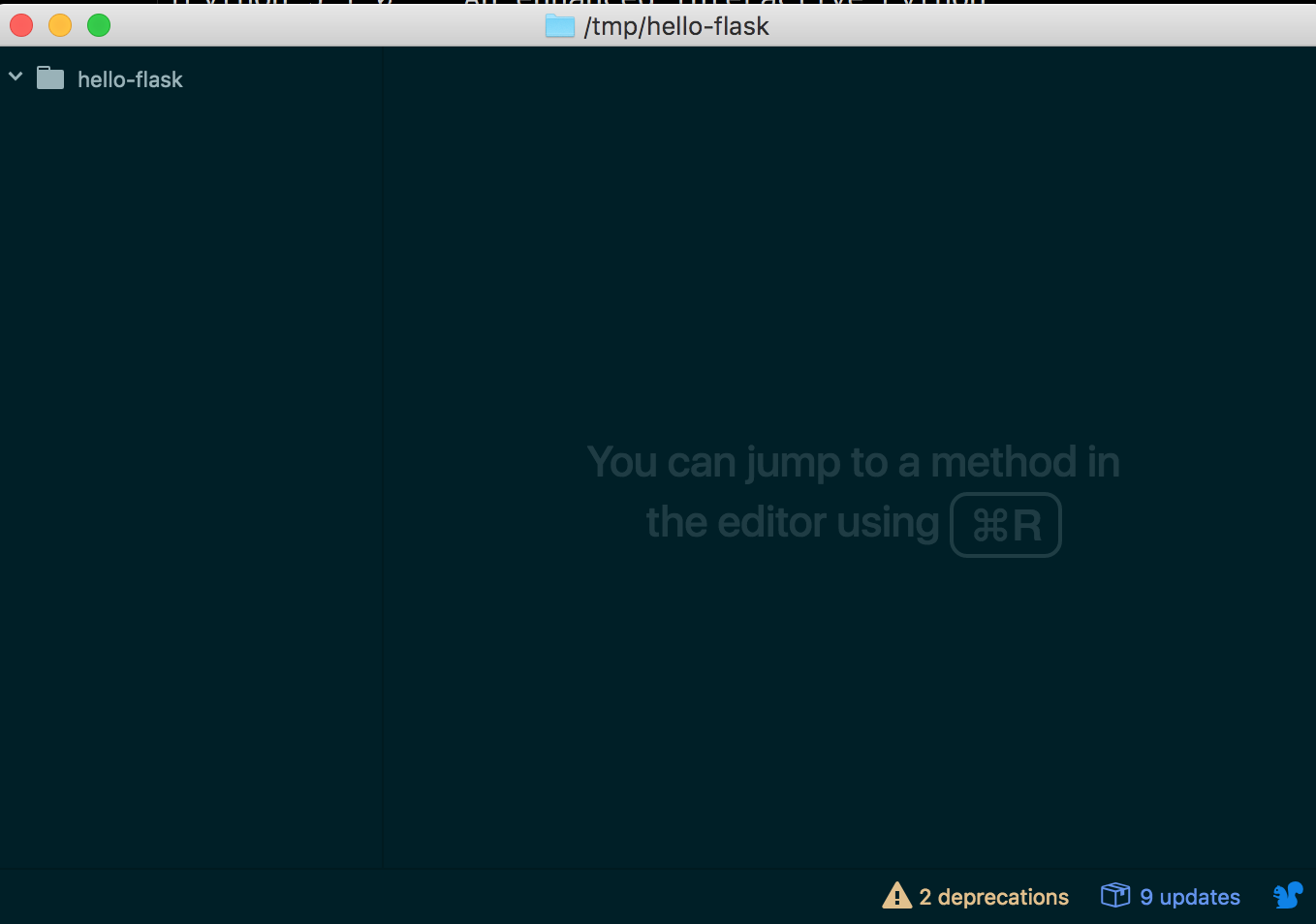
Creating hello-flask/app.py
¶
Let’s create our first file in the project folder. From Atom, I find the easiest thing to do is to right-click on the project folder name, e.g. /tmp/hello-flask
to bring up the context-menu and select the command to make a new file:
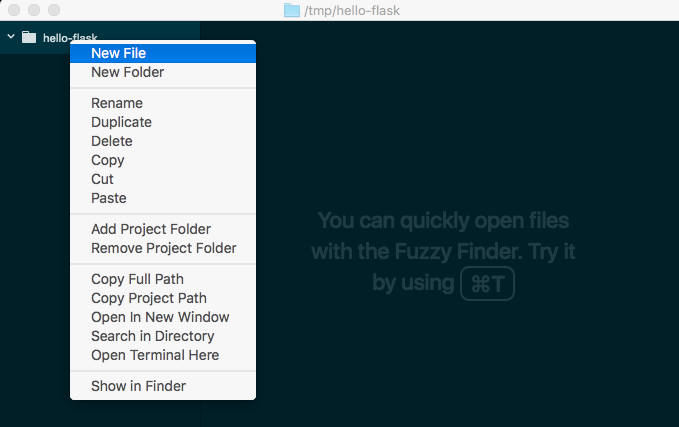
Name the new file, app.py
.
The new file should appear as a child of your main folder:
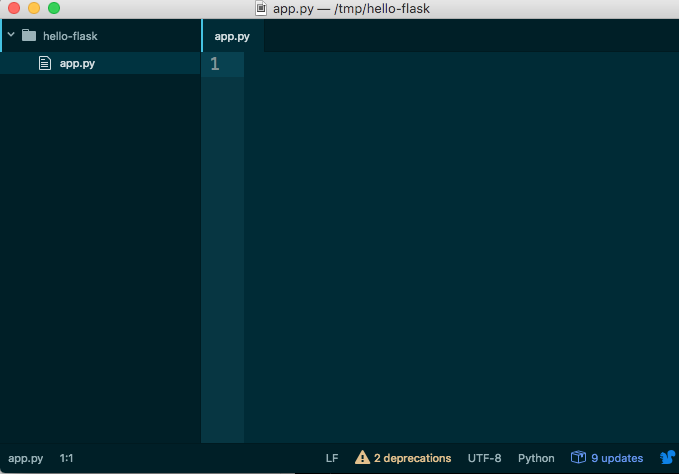
Writing hello-flask/app.py
¶
Now, let’s add Flask code. I know I’ve been saying never to write any Python code that you haven’t tested, line by line, in the interactive shell. But for code that defines an entire application – well, it doesn’t make much sense to paste it straight into iPython. And if you’re new to web development, you don’t even know what to test, or what a web application does.
So for now, just type out this code into app.py
. I highly recommend not copying and pasting it, but typing it out manually, which slows you down and makes you realize that all of this strange code – minus just one line – is Python you’ve written before:
from flask import Flask
application = Flask(__name__)
@application.route('/')
def homepage():
return "Hello world!"
if __name__ == '__main__':
application.run(debug=True, use_reloader=True)
Note
What is @
, and is a Python decorator?
The one alien piece of code, the line that begins with the @
sign, denotes a Python decorator, a concept we haven’t learned nor will we cover by the end of the course.
For the purposes of this project, you only have to see that it’s used in the same context, over and over, in a Flask web application, in a predictable pattern.
Running app.py as a local web application¶
The app.py
script is our web application – I already told you it’d be just one file. How do we “run” it as a web app?
Go to your command line and run it as any other Python script:
$ python app.py
You’ll get some output like this:
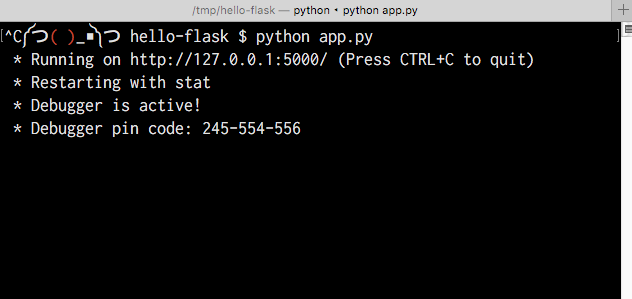
And curiously enough, the script will not “end”. That is, this web app is meant to run “forever”, or at least until your computer shuts off or you hit Ctrl-C.
But before we shut down our web app, let’s visit it via our browser.
Visiting our local web application¶
The output of running app.py
should contain a message like this:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
That URL looks like a web address, right?
Where does it go? As it turns out, the address of 127.0.0.1
is used to refer to the local computer? The 5000
part means “port 5000” – something we isn’t important to us other than being part of the full URL.
So visiting that address in the browser will result in this:
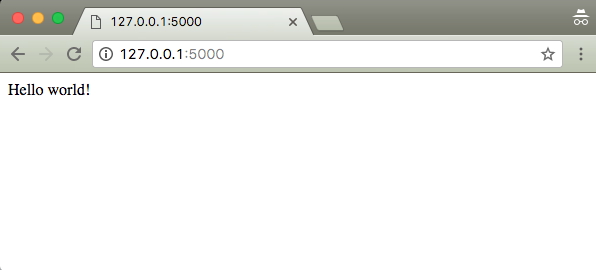
Shutting down our web app¶
Now let’s “kill” our web app, or at least the computational process that is letting it run forever in the background. Easiest way to do this is to switch back your Terminal in which the app is running and hit: Ctrl-C
What happens when we visit the address for our web app? Try it:
Welcome to one of many kinds of errors – in this case, the webserver simply isn’t running – that we prepare for when dealing with web applications:
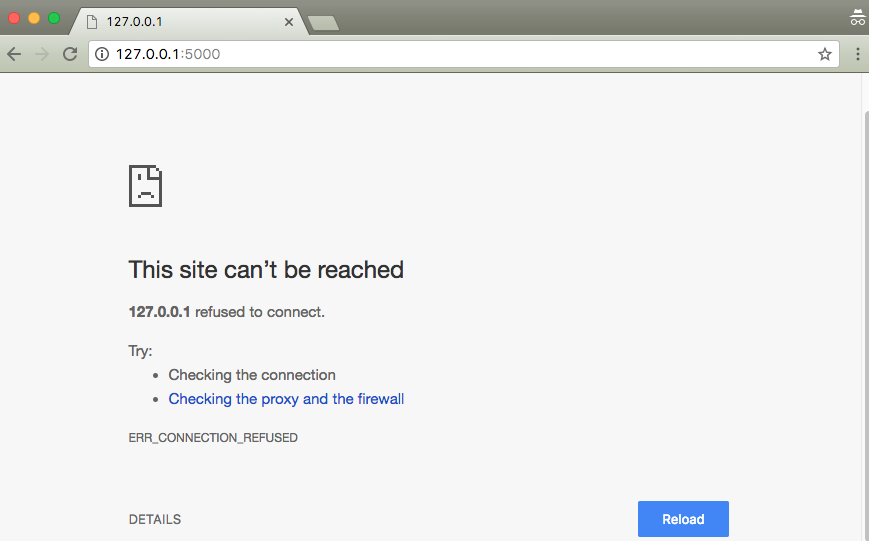
Routes in our web application¶
Go into the app.py
file and look for these lines:
@application.route('/')
def homepage():
return "Hello world!"
Here’s the point: the @application
object has a route
method that defines what URL the application should respond to By default, our app responds to this URL:
Let’s change it up. That value for the route
method is just a string, which means we should be able to alter the lines like this:
@application.route('/foobarbaby')
def homepage():
return "Hello messed upworld!"
Save app.py
and restart the web application:
$ python app.py
Then visit this URL:
Your browser should respond predictably:
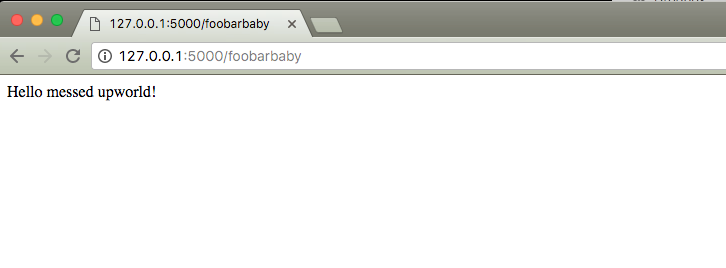
But what happen the original, ordinary homepage route, i.e.
Visit it yourself. Now you’ll get a “Not Found” error:
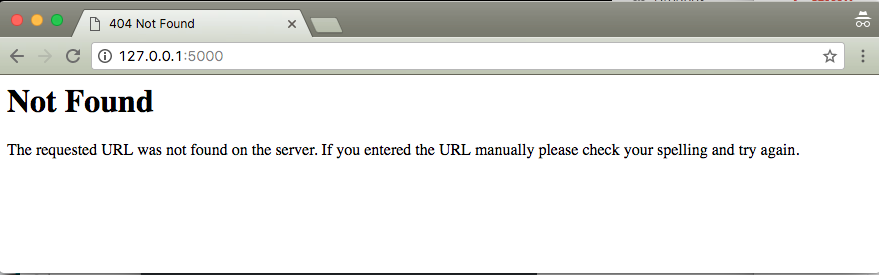
Congratulations, you’ve just made your first 404 response!
Why did going to the purported root/homepage of 127.0.0.1 result in a 404? Because we altered the homepage
route to have a different string pattern:
@application.route('/foobarbaby')
def homepage():
return "Hello messed upworld!"
See if you can figure out how to make a new route so that the web app responds to /
and /foobarbaby
.
Here’s an example of a tutorial Flask app with multiple (and dynamic) routes: Creating Multiple Routes and Dynamic Content in Flask
Dynamic routes¶
Like placecage.com, we want our simple web app to respond to different URLs, not just hard-coded strings /
and /foobarbaby
This is where we use the URL Route Registration feature of Flask:
Using this feature, we are able to define a dynamic route for our application too look for, and then to parse the dynamic parts as arguments to a function. Here is app.py
all over again, except with a response to the /
route, and to: /hello/<name>
from flask import Flask
application = Flask(__name__)
@application.route('/')
def homepage():
return "Hello world!"
@application.route('/hello/<your_name>')
def hello(your_name):
return "Hello {}!!!".format(your_name)
if __name__ == '__main__':
application.run(debug=True, use_reloader=True)
Restart the app. What happens when you go to the following URLs?
That’s an App!¶
Congratulations. You’ve built a web application. And you’ve built something flexible enough to connect something, say a bot script, to a URL, in such a way that visiting a URL will trigger that bot’s action.
At this point, I highly recommend going through the First News App tutorial from beginning to...everything before the Hello Internet heading.
If you’ve never done web app development, you’ll see how data is used to create a news application. And how HTML is a text string but still kind of different that rendering 'hello world'
References¶
- Getting Started with Github
- Introduction to Simple Web Applications With Flask - A scattered collection of Flask/web-dev examples from my Computational Journalism course last year.
- Hello Tiny Flask App - “How to make the simplest of Python web applications.” (in retrospect, it could be simpler)
- Flask documentation
- Flask docs on URL Route Registrations